Segment
Segment is a powerful tool that allows you to track the usage of your customers, providing valuable insights that can help you make data-driven decisions. This data can be sent to Lago, our usage-based billing platform, to automate your billing process and ensure accurate invoicing for your customers.
Here’s a step-by-step guide to help you get started:
Prerequisites
In Lago:
- Create a Lago organization to manage your billing and invoicing;
- Create a Billable metric to track the usage of your customers;
- Create a Plan and price the above billable metric to determine the billing rates for your customers; and
- Create a Customer and assign the Plan.
In Segment:
- Create a Segment account;
- Create a data source (ideally, product usage of your customer);
Send usage from Segment to Lago
Create a function
To accomplish this, you’ll need to create a custom Function in Segment. This can be done by following these simple steps:
- Navigate to the Catalog in Segment;
- Click the Functions tab to access the custom Functions feature;
- Choose to create your first function and follow the prompts to set it up.
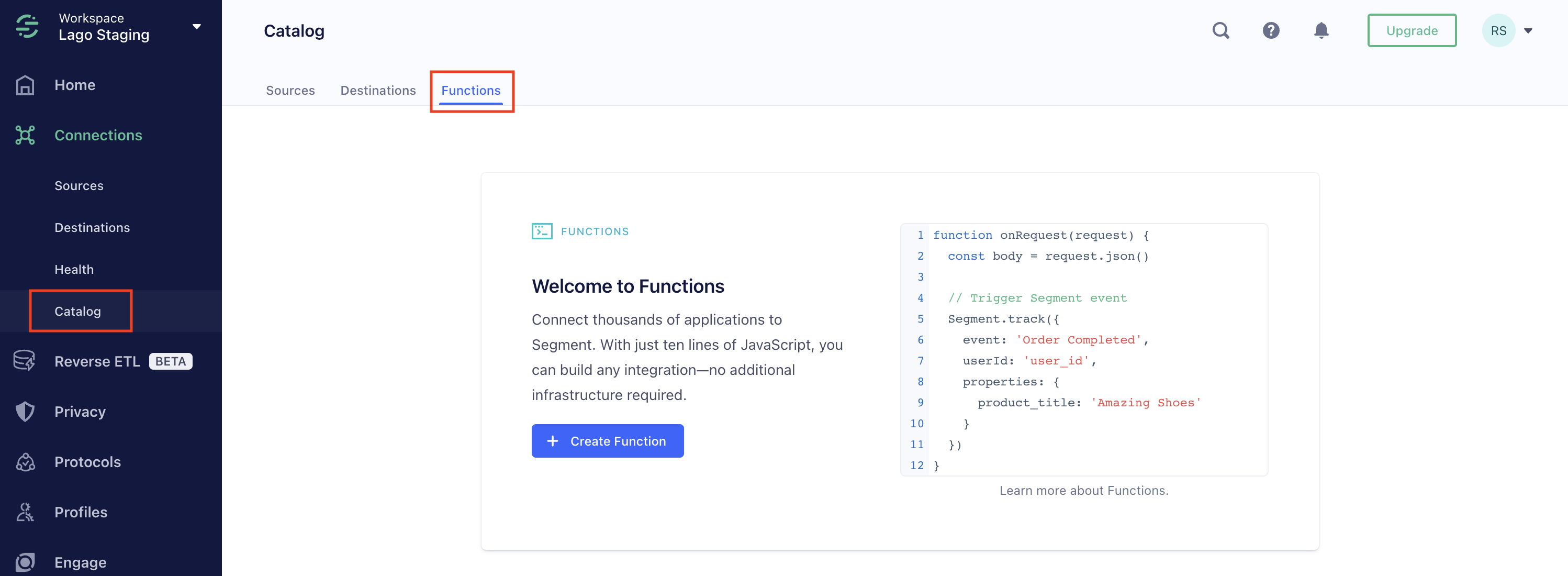
Segment Functions
Use the Destination function
Make sure to select the Destination function, as you want to send data from Segment to Lago.
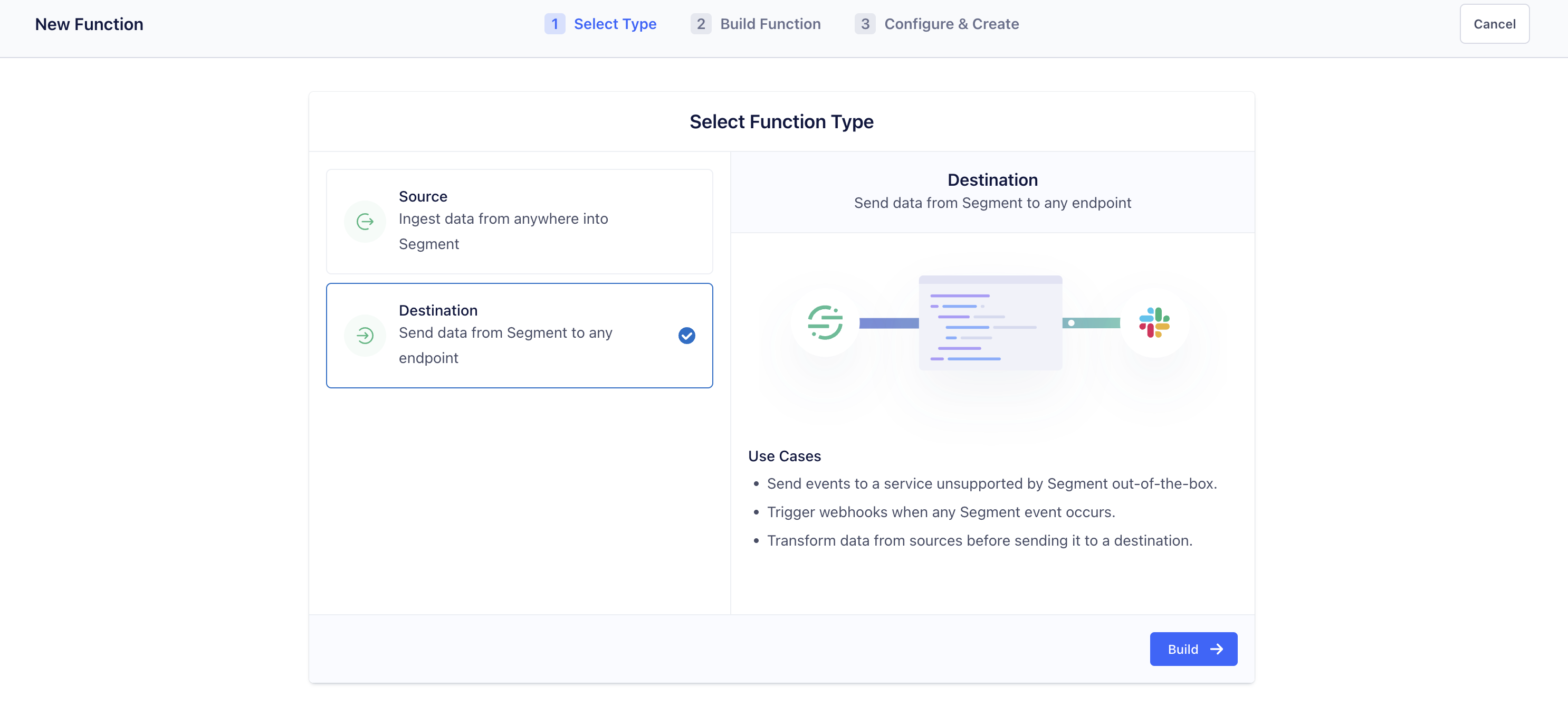
Destinations function
Post Request to Lago events
To successfully integrate Lago with Segment, you’ll need to replace the pre-written functions in the code editor with the following code. This example function, written by the Lago team, will catch a Track event from Segment, define the targeted endpoint (events) in Lago, build the body of the request, and finally post the event.
// Running everytime a Track call is made on Segment
async function onTrack(event, settings) {
// events endpoint to reach
const endpoint = 'https://api.getlago.com/api/v1/events';
// body of the event following Lago documentation
const body = {
event: {
transactionId: event.messageId,
externalCustomerId: event.userId,
code: event.event,
properties: {
invoiceId: event.properties.invoice_id
}
}
};
// Post event
const response = await fetch(endpoint, {
method: 'POST',
headers: {
'Authorization': `Bearer ${settings.api}`,
'Content-Type': 'application/json'
},
body: JSON.stringify(body)
});
return response.json();
}
This function can be adapted with Identify, Group or Page events. Please, refer to Segment’s documentation for all available actions.
The body
structure of the event depends on your use case. Please adapt it if needed (ie: remove or add properties). You can also add conditions if you want to send data to Lago only on specific events.
Use the test mode editor
By using a sample event, you can preview the incoming data fetched from a Segment event. This will help you post a request for existing data or debug.
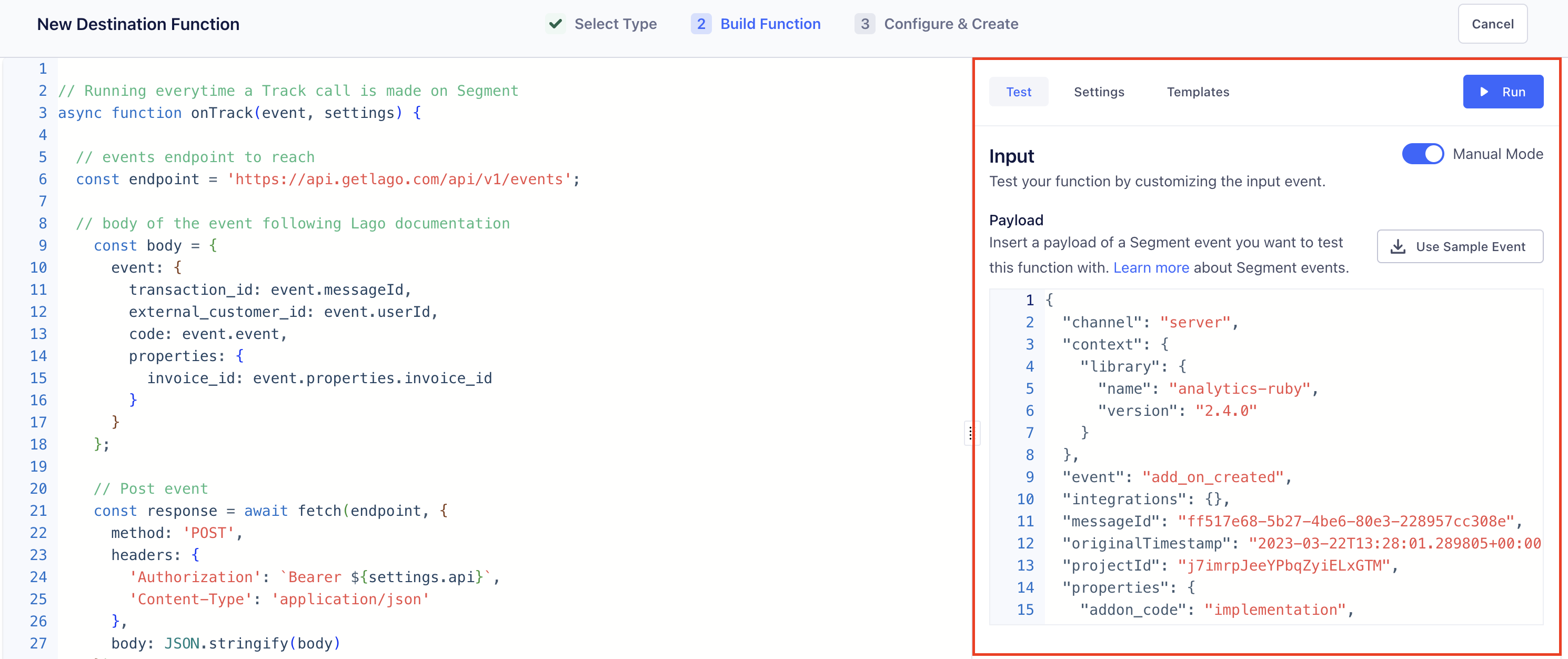
Segment Sample Events
Hide sensitive data
Let’s take back the example from the code written above. We decided to hide the API Key and mark it as sensitive information. By setting this as a variable, you make sure not to hard code your private key in the function.
To create Settings variables:
- Go to the Settings tab;
- Add a new Setting;
- Define a Name and a Label for this Settings;
- Define it as Required or Optional; and
- Mark is as Sensitive or not.
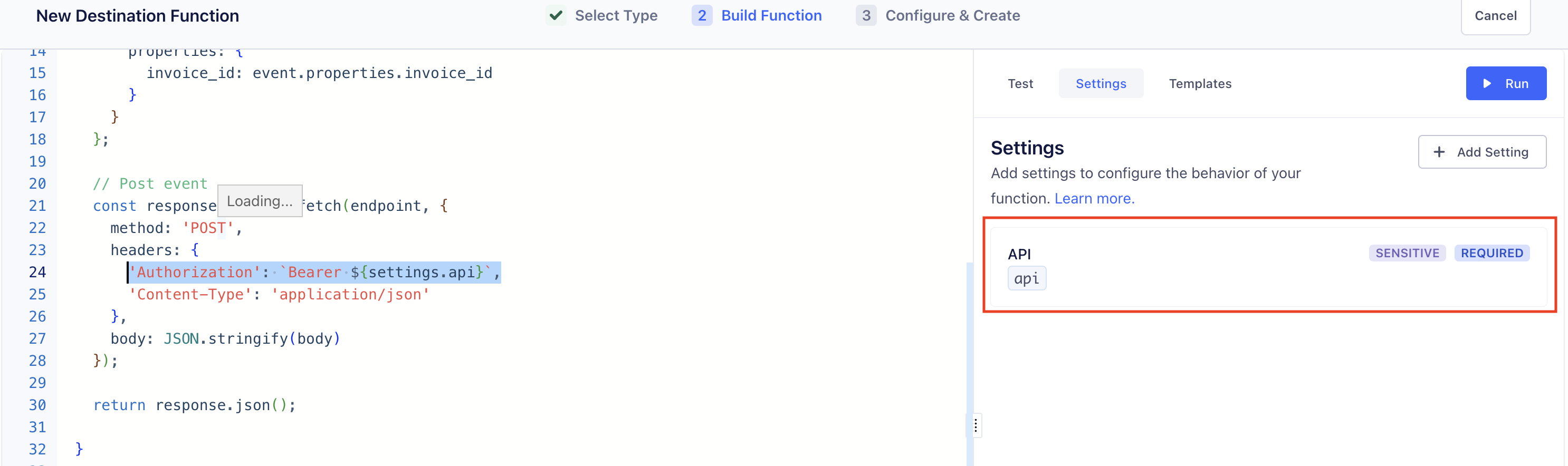
Hide sensitive data with settings variables
Send usage events to Lago
By running the function in Segment, this will send a test usage to Lago events. You can retrieve this event in the events list. By finalizing the setup in Segment, the function will be automatically triggered based on your defined behavior
Segment to Lago - demo video
If easier, please find a demo video explaining the full setup of custom functions to send event from Segment.com to Lago.
Was this page helpful?